找到图中所有封闭游走的算法,适用于给定的顶点
今日不肯埋头,明日何以抬头!每日一句努力自己的话哈哈~哈喽,今天我将给大家带来一篇《找到图中所有封闭游走的算法,适用于给定的顶点》,主要内容是讲解等等,感兴趣的朋友可以收藏或者有更好的建议在评论提出,我都会认真看的!大家一起进步,一起学习!
我有一个无向图,没有多个/平行边,并且每个边都用距离加权。我希望在图表中找到某个最小和最大总距离之间的封闭行走。我还希望能够对步行中重复距离的数量设置限制,例如,如果将 10 的边遍历两次,则重复距离为 10。将重复距离设置为 0 应该会发现闭合图中的路径(与行走相对)。最后,虽然找到所有封闭步道会很好,但我不确定这对于存在数千/数百万条潜在路线的较大图表是否可行,因为我相信这将花费指数时间。因此,我目前正在尝试根据启发式函数找到一些最佳的封闭行走,以便算法在合理的时间内完成。
编辑:大致性能要求 - 我用来测试的图当前有 17,070 个节点和 23,026 个边。生成的循环包含大约 50 个边,查找时间约为 20 秒,但是我希望找到更长距离的循环(~100 个边),理想情况下也在 <1 分钟的时间范围内。
这是我当前的方法:
graph.go:
package graph import ( "fmt" "github.com/bgadrian/data-structures/priorityqueue" ) // a graph struct is a collection of nodes and edges represented as an adjacency list. // each edge has a weight (float64). type edge struct { heuristicvalue int // lower heuristic = higher priority distance float64 } type graph struct { edges map[int]map[int]edge // adjacency list, accessed like edges[fromid][toid] } func (g *graph) findallloops(start int, mindistance float64, maxdistance float64, maxrepeated float64, routescap int) []route { // find all loops that start and end at node start. // a loop is a closed walk through the graph. loops := []route{} loopcount := 0 queue, _ := priorityqueue.newhierarchicalheap(100, 0, 100, false) queue.enqueue(*newroute(start), 0) size := 1 distancetostart := getdistancetostart(g, start) for size > 0 { // pop the last element from the stack temp, _ := queue.dequeue() size-- route := temp.(route) // get the last node from the route lastnode := route.lastnode() // if the route is too long or has too much repeated distance, // don't bother exploring it further if route.distance + distancetostart[route.lastnode()] > maxdistance || route.repeateddistance > maxrepeated { continue } // now we need to efficiently check if the total repeated distance is too long // if the last node is the start node and the route is long enough, add it to the list of loops if lastnode == start && route.distance >= mindistance && route.distance <= maxdistance { loops = append(loops, route) loopcount++ if loopcount >= routescap { return loops } } // add all the neighbours of the last node to the stack for neighbour := range g.edges[lastnode] { // newroute will be a copy of the current route, but with the new node added newroute := route.withaddednode(neighbour, g.edges[lastnode][neighbour]) queue.enqueue(newroute, newroute.heuristic()) size++ } } return loops }
路线.go:
package graph import ( "fmt" ) // A route is a lightweight struct describing a route // It is stored as a sequence of nodes (ints, which are the node ids) // and a distance (float64) // It also counts the total distance that is repeated (going over the same // edge twice) // To do this, it stores a list of edges that have been traversed before type Route struct { Nodes []int Distance float64 Repeated []IntPair // two ints corresponding to the nodes, orderedd by (smallest, largest) RepeatedDistance float64 Heuristic int // A heuristic for the type of Routes we would like to generate LastWay int } type IntPair struct { A int B int } // WithAddedNode adds a node to the route func (r *Route) WithAddedNode(node int, edge Edge) Route { newRoute := Route{Nodes: make([]int, len(r.Nodes) + 1), Distance: r.Distance, Repeated: make([]IntPair, len(r.Repeated), len(r.Repeated) + 1), RepeatedDistance: r.RepeatedDistance, Ways: r.Ways, LastWay: r.LastWay} copy(newRoute.Nodes, r.Nodes) copy(newRoute.Repeated, r.Repeated) newRoute.Nodes[len(r.Nodes)] = node newRoute.Distance += edge.Distance // Get an IntPair where A is the smaller node id and B is the larger var pair IntPair if newRoute.Nodes[len(newRoute.Nodes)-2] < node { pair = IntPair{newRoute.Nodes[len(newRoute.Nodes)-2], node} } else { pair = IntPair{node, newRoute.Nodes[len(newRoute.Nodes)-2]} } // Check if the edge has been traversed before found := false for _, p := range newRoute.Repeated { if p == pair { newRoute.RepeatedDistance += edge.Distance found = true break } } if !found { newRoute.Repeated = append(newRoute.Repeated, pair) } // Current heuristic sums heuristics of edges but this may change in the future newRoute.Heuristic += edge.HeuristicValue return newRoute } func (r *Route) Heuristic() int { return r.Heuristic } func (r *Route) LastNode() int { return r.Nodes[len(r.Nodes)-1] }
以下是我目前为加速算法所做的事情:
- 不是查找所有循环,而是根据给定的启发式查找前几个循环。
- 修剪图表,尽可能删除几乎所有 2 阶节点,并用权重 = 原始两条边之和的单条边替换它们。
- 使用切片来存储重复的边,而不是使用地图。
- 使用 dijkstra 算法找到从起始节点到每个其他节点的最短距离。如果一条路线无法在这段距离内返回,请立即丢弃它。 (算法实现的代码未包含在帖子中,因为它只占运行时间的约 4%)
根据 go 分析器,route.go 中的 withaddednode 方法占运行时的 68%,该方法的时间大致均匀地分布在 runtime.memmove 和 runtime.makeslice (调用 runtime.mallocgc)之间。本质上,我相信大部分时间都花在复制路由上。我将非常感谢任何有关如何改进该算法的运行时间或任何可能的替代方法的反馈。如果我可以提供任何其他信息,请告诉我。非常感谢!
正确答案
查找两个顶点之间的所有路径的标准算法是:
- 应用 dijkstra 寻找最便宜的路径
- 增加路径中链接的成本
- 重复直到找不到新路径
在您的情况下,您希望路径返回到起始顶点。可以轻松修改算法来做到这一点
- 将起始顶点 s 分成两部分:s1 和 s2。
- 在连接到 s 的顶点上循环 v。
- 在 s1 和 v 之间添加零成本边
- 在 s2 和 v 之间添加零成本边
- 删除 s 及其边缘。
- 运行标准算法来查找 s1 和 s2 之间的所有路径
您需要修改 dijkstra 代码以考虑其他限制 - 最大长度和“启发式”
性能
尽管您说您担心大型图表的性能,但您没有给出所需的性能。我会尝试提供一个估计。
我对 go 的性能一无所知,所以我假设它与我熟悉的 c++ 相同。
以下是 c++ 的一些性能测量:
运行 dijkstra 算法的运行时间,以在从 https://dyngraphlab.github.io/。使用 graphex 应用程序运行 3 次的最长结果。
Vertex Count | Edge Count | Run time ( secs ) |
---|---|---|
10,000 | 50,000 | 0.3 |
145,000 | 2,200,000 | 40 |
今天关于《找到图中所有封闭游走的算法,适用于给定的顶点》的内容介绍就到此结束,如果有什么疑问或者建议,可以在golang学习网公众号下多多回复交流;文中若有不正之处,也希望回复留言以告知!
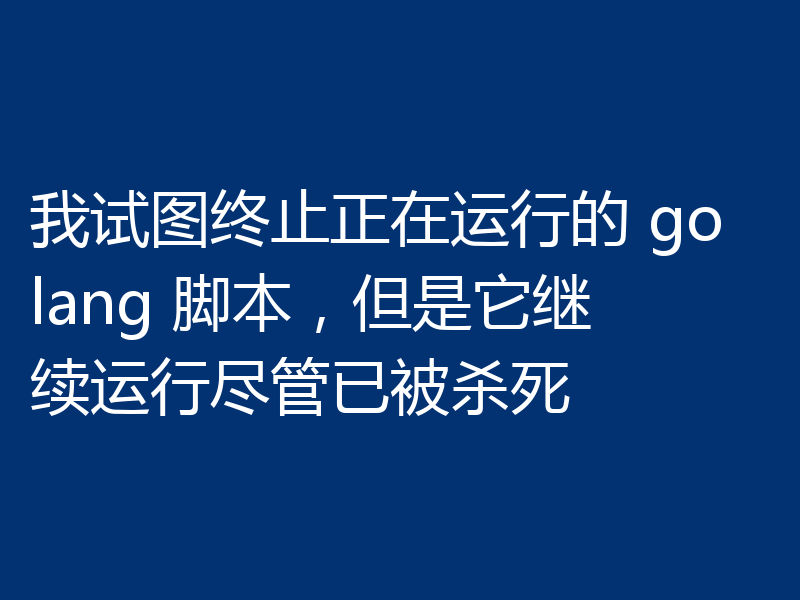
- 上一篇
- 我试图终止正在运行的 golang 脚本,但是它继续运行尽管已被杀死
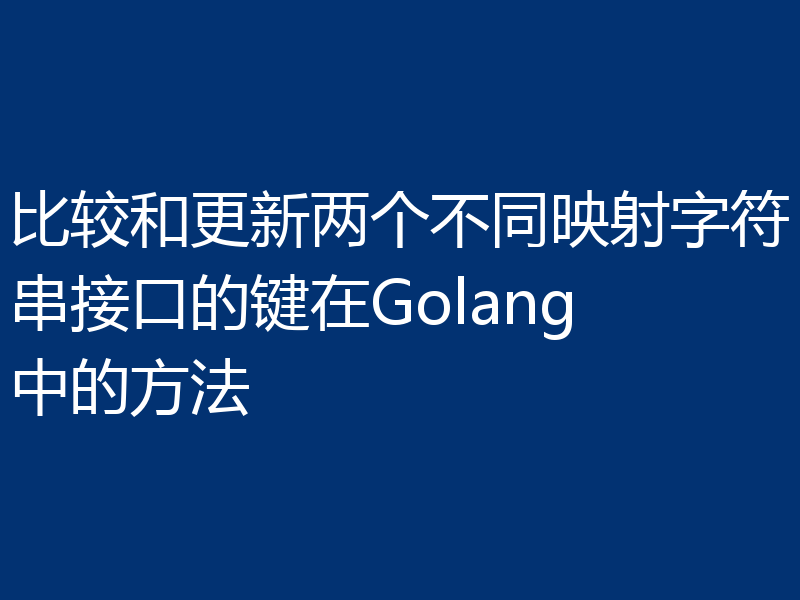
- 下一篇
- 比较和更新两个不同映射字符串接口的键在Golang中的方法
-
- Golang · Go问答 | 1年前 |
- 在读取缓冲通道中的内容之前退出
- 139浏览 收藏
-
- Golang · Go问答 | 1年前 |
- 戈兰岛的全球 GOPRIVATE 设置
- 204浏览 收藏
-
- Golang · Go问答 | 1年前 |
- 如何将结构作为参数传递给 xml-rpc
- 325浏览 收藏
-
- Golang · Go问答 | 1年前 |
- 如何用golang获得小数点以下两位长度?
- 477浏览 收藏
-
- Golang · Go问答 | 1年前 |
- 如何通过 client-go 和 golang 检索 Kubernetes 指标
- 486浏览 收藏
-
- Golang · Go问答 | 1年前 |
- 将多个“参数”映射到单个可变参数的习惯用法
- 439浏览 收藏
-
- Golang · Go问答 | 1年前 |
- 将 HTTP 响应正文写入文件后出现 EOF 错误
- 357浏览 收藏
-
- Golang · Go问答 | 1年前 |
- 结构中映射的匿名列表的“复合文字中缺少类型”
- 352浏览 收藏
-
- Golang · Go问答 | 1年前 |
- NATS Jetstream 的性能
- 101浏览 收藏
-
- Golang · Go问答 | 1年前 |
- 如何将复杂的字符串输入转换为mapstring?
- 440浏览 收藏
-
- Golang · Go问答 | 1年前 |
- 相当于GoLang中Java将Object作为方法参数传递
- 212浏览 收藏
-
- Golang · Go问答 | 1年前 |
- 如何确保所有 goroutine 在没有 time.Sleep 的情况下终止?
- 143浏览 收藏
-
- 前端进阶之JavaScript设计模式
- 设计模式是开发人员在软件开发过程中面临一般问题时的解决方案,代表了最佳的实践。本课程的主打内容包括JS常见设计模式以及具体应用场景,打造一站式知识长龙服务,适合有JS基础的同学学习。
- 542次学习
-
- GO语言核心编程课程
- 本课程采用真实案例,全面具体可落地,从理论到实践,一步一步将GO核心编程技术、编程思想、底层实现融会贯通,使学习者贴近时代脉搏,做IT互联网时代的弄潮儿。
- 508次学习
-
- 简单聊聊mysql8与网络通信
- 如有问题加微信:Le-studyg;在课程中,我们将首先介绍MySQL8的新特性,包括性能优化、安全增强、新数据类型等,帮助学生快速熟悉MySQL8的最新功能。接着,我们将深入解析MySQL的网络通信机制,包括协议、连接管理、数据传输等,让
- 497次学习
-
- JavaScript正则表达式基础与实战
- 在任何一门编程语言中,正则表达式,都是一项重要的知识,它提供了高效的字符串匹配与捕获机制,可以极大的简化程序设计。
- 487次学习
-
- 从零制作响应式网站—Grid布局
- 本系列教程将展示从零制作一个假想的网络科技公司官网,分为导航,轮播,关于我们,成功案例,服务流程,团队介绍,数据部分,公司动态,底部信息等内容区块。网站整体采用CSSGrid布局,支持响应式,有流畅过渡和展现动画。
- 484次学习
-
- 茅茅虫AIGC检测
- 茅茅虫AIGC检测,湖南茅茅虫科技有限公司倾力打造,运用NLP技术精准识别AI生成文本,提供论文、专著等学术文本的AIGC检测服务。支持多种格式,生成可视化报告,保障您的学术诚信和内容质量。
- 103次使用
-
- 赛林匹克平台(Challympics)
- 探索赛林匹克平台Challympics,一个聚焦人工智能、算力算法、量子计算等前沿技术的赛事聚合平台。连接产学研用,助力科技创新与产业升级。
- 112次使用
-
- 笔格AIPPT
- SEO 笔格AIPPT是135编辑器推出的AI智能PPT制作平台,依托DeepSeek大模型,实现智能大纲生成、一键PPT生成、AI文字优化、图像生成等功能。免费试用,提升PPT制作效率,适用于商务演示、教育培训等多种场景。
- 122次使用
-
- 稿定PPT
- 告别PPT制作难题!稿定PPT提供海量模板、AI智能生成、在线协作,助您轻松制作专业演示文稿。职场办公、教育学习、企业服务全覆盖,降本增效,释放创意!
- 110次使用
-
- Suno苏诺中文版
- 探索Suno苏诺中文版,一款颠覆传统音乐创作的AI平台。无需专业技能,轻松创作个性化音乐。智能词曲生成、风格迁移、海量音效,释放您的音乐灵感!
- 110次使用
-
- GoLand调式动态执行代码
- 2023-01-13 502浏览
-
- 用Nginx反向代理部署go写的网站。
- 2023-01-17 502浏览
-
- Golang取得代码运行时间的问题
- 2023-02-24 501浏览
-
- 请问 go 代码如何实现在代码改动后不需要Ctrl+c,然后重新 go run *.go 文件?
- 2023-01-08 501浏览
-
- 如何从同一个 io.Reader 读取多次
- 2023-04-11 501浏览