如何在两个 goroutine 之间共享一个 HTTP 请求实例?
今日不肯埋头,明日何以抬头!每日一句努力自己的话哈哈~哈喽,今天我将给大家带来一篇《如何在两个 goroutine 之间共享一个 HTTP 请求实例?》,主要内容是讲解等等,感兴趣的朋友可以收藏或者有更好的建议在评论提出,我都会认真看的!大家一起进步,一起学习!
我现在有一些代码可以发出 3 个请求来填充 3 个变量。两个请求是相同的。我想在两个不同的函数之间共享一个http请求(在现实世界中,这些函数被分成两个不同的模块)。
让我根据比现实世界中简单得多的示例来描述我所遇到的问题。
目前,我有以下主要功能和 post 数据结构:
type post struct { id int `json:"id"` title string `json:"title"` userid int `json:"userid"` iscompleted bool `json:"completed"` } func main() { var wg sync.waitgroup fmt.println("hello, world.") wg.add(3) var firstpostid int var secondpostid int var secondpostname string go func() { firstpostid = getfirstpostid() defer wg.done() }() go func() { secondpostid = getsecondpostid() defer wg.done() }() go func() { secondpostname = getsecondpostname() defer wg.done() }() wg.wait() fmt.println("first post id is", firstpostid) fmt.println("second post id is", secondpostid) fmt.println("second post title is", secondpostname) }
有 3 个 goroutine,所以我有 3 个并发请求,我使用 sync.workgroup
同步所有内容。以下代码是请求的实现:
func makerequest(url string) post { resp, err := http.get(url) if err != nil { // handle error } defer resp.body.close() body, err := ioutil.readall(resp.body) var post post json.unmarshal(body, &post) return post } func makefirstpostrequest() post { return makerequest("https://jsonplaceholder.typicode.com/todos/1") } func makesecondpostrequest() post { return makerequest("https://jsonplaceholder.typicode.com/todos/2") }
以下是从获取的帖子中提取所需信息的函数的实现:
func getfirstpostid() int { var result = makefirstpostrequest() return result.id } func getsecondpostid() int { var result = makesecondpostrequest() return result.id } func getsecondpostname() string { var result = makesecondpostrequest() return result.title }
所以,目前我有 3 个并发请求,这工作得很好。问题是我不希望 2 个完全相同的单独 http 请求来获取第二篇文章。一个就足够了。所以,我想要实现的是 post 1 和 post 2 的 2 个并发请求。我希望第二次调用 makesecondpostrequest
不是创建新的 http 请求,而是共享现有的请求(由第一次调用发送)。
我怎样才能实现这个目标?
注意:例如,以下代码是如何使用 javascript 来完成此操作的。
let promise = null; function makeRequest() { if (promise) { return promise; } return promise = fetch('https://jsonplaceholder.typicode.com/todos/1') .then(result => result.json()) // clean up cache variable, so any next request in the future will be performed again .finally(() => (promise = null)) } function main() { makeRequest().then((post) => { console.log(post.id); }); makeRequest().then((post) => { console.log(post.title); }); } main();
解决方案
虽然您可以将诸如 promise 之类的东西放在一起,但在这种情况下没有必要。
您的代码是以过程方式编写的。您已经编写了非常具体的函数,这些函数从 post
中提取特定的小部分并丢弃其余部分。相反,请将您的 post
放在一起。
package main import( "fmt" "encoding/json" "net/http" "sync" ) type post struct { id int `json:"id"` title string `json:"title"` userid int `json:"userid"` iscompleted bool `json:"completed"` } func fetchpost(id int) post { url := fmt.sprintf("https://jsonplaceholder.typicode.com/todos/%v", id) resp, err := http.get(url) if err != nil { panic("http error") } defer resp.body.close() // it's more efficient to let json decoder handle the io. var post post decoder := json.newdecoder(resp.body) err = decoder.decode(&post) if err != nil { panic("decoding error") } return post } func main() { var wg sync.waitgroup wg.add(2) var firstpost post var secondpost post go func() { firstpost = fetchpost(1) defer wg.done() }() go func() { secondpost = fetchpost(2) defer wg.done() }() wg.wait() fmt.println("first post id is", firstpost.id) fmt.println("second post id is", secondpost.id) fmt.println("second post title is", secondpost.title) }
现在您可以缓存帖子,而不是缓存响应。我们可以通过添加 postmanager 来处理获取和缓存帖子来做到这一点。
请注意,普通的 map
对于并发使用并不安全,因此我们使用 sync.Map 作为缓存。
type postmanager struct { sync.map } func (pc *postmanager) fetch(id int) post { post, ok := pc.load(id) if ok { return post.(post) } post = pc.fetchpost(id) pc.store(id, post) return post.(post) } func (pc *postmanager) fetchpost(id int) post { url := fmt.sprintf("https://jsonplaceholder.typicode.com/todos/%v", id) resp, err := http.get(url) if err != nil { panic("http error") } defer resp.body.close() // it's more efficient to let json decoder handle the io. var post post decoder := json.newdecoder(resp.body) err = decoder.decode(&post) if err != nil { panic("decoding error") } return post }
postmanager
方法必须采用指针接收器以避免复制 sync.map
内的互斥体。
我们使用 postmanager,而不是直接获取帖子。
func main() { var postmanager postmanager var wg sync.waitgroup wg.add(2) var firstpost post var secondpost post go func() { firstpost = postmanager.fetch(1) defer wg.done() }() go func() { secondpost = postmanager.fetch(2) defer wg.done() }() wg.wait() fmt.println("first post id is", firstpost.id) fmt.println("second post id is", secondpost.id) fmt.println("second post title is", secondpost.title) }
通过使用 conditional requests 检查缓存的 post 是否已更改,可以改进 postmanager 的缓存。
它的锁定也可以得到改进,因为可以同时获取同一篇文章。我们可以使用 singleflight
修复此问题,以允许一次仅对具有给定 id 的 fetchpost
进行一次调用。
type PostManager struct { group singleflight.Group cached sync.Map } func (pc *PostManager) Fetch(id int) Post { post,ok := pc.cached.Load(id) if !ok { // Multiple calls with the same key at the same time will only run the code once, but all calls get the result. post, _, _ = pc.group.Do(strconv.Itoa(id), func() (interface{}, error) { post := pc.fetchPost(id) pc.cached.Store(id, post) return post, nil }) } return post.(Post) }
好了,本文到此结束,带大家了解了《如何在两个 goroutine 之间共享一个 HTTP 请求实例?》,希望本文对你有所帮助!关注golang学习网公众号,给大家分享更多Golang知识!
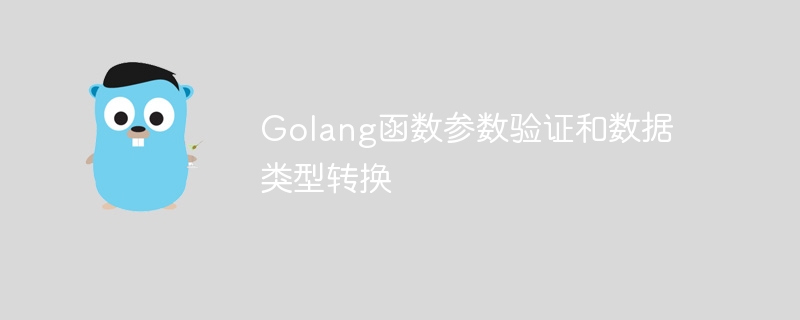
- 上一篇
- Golang函数参数验证和数据类型转换
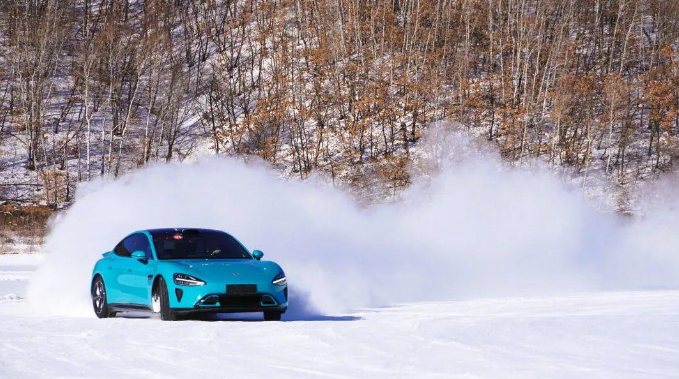
- 下一篇
- 小米新车效应炸裂,车企大降价应对,谁将成最大赢家?
-
- Golang · Go问答 | 1年前 |
- 在读取缓冲通道中的内容之前退出
- 139浏览 收藏
-
- Golang · Go问答 | 1年前 |
- 戈兰岛的全球 GOPRIVATE 设置
- 204浏览 收藏
-
- Golang · Go问答 | 1年前 |
- 如何将结构作为参数传递给 xml-rpc
- 325浏览 收藏
-
- Golang · Go问答 | 1年前 |
- 如何用golang获得小数点以下两位长度?
- 477浏览 收藏
-
- Golang · Go问答 | 1年前 |
- 如何通过 client-go 和 golang 检索 Kubernetes 指标
- 486浏览 收藏
-
- Golang · Go问答 | 1年前 |
- 将多个“参数”映射到单个可变参数的习惯用法
- 439浏览 收藏
-
- Golang · Go问答 | 1年前 |
- 将 HTTP 响应正文写入文件后出现 EOF 错误
- 357浏览 收藏
-
- Golang · Go问答 | 1年前 |
- 结构中映射的匿名列表的“复合文字中缺少类型”
- 352浏览 收藏
-
- Golang · Go问答 | 1年前 |
- NATS Jetstream 的性能
- 101浏览 收藏
-
- Golang · Go问答 | 1年前 |
- 如何将复杂的字符串输入转换为mapstring?
- 440浏览 收藏
-
- Golang · Go问答 | 1年前 |
- 相当于GoLang中Java将Object作为方法参数传递
- 212浏览 收藏
-
- Golang · Go问答 | 1年前 |
- 如何确保所有 goroutine 在没有 time.Sleep 的情况下终止?
- 143浏览 收藏
-
- 前端进阶之JavaScript设计模式
- 设计模式是开发人员在软件开发过程中面临一般问题时的解决方案,代表了最佳的实践。本课程的主打内容包括JS常见设计模式以及具体应用场景,打造一站式知识长龙服务,适合有JS基础的同学学习。
- 542次学习
-
- GO语言核心编程课程
- 本课程采用真实案例,全面具体可落地,从理论到实践,一步一步将GO核心编程技术、编程思想、底层实现融会贯通,使学习者贴近时代脉搏,做IT互联网时代的弄潮儿。
- 508次学习
-
- 简单聊聊mysql8与网络通信
- 如有问题加微信:Le-studyg;在课程中,我们将首先介绍MySQL8的新特性,包括性能优化、安全增强、新数据类型等,帮助学生快速熟悉MySQL8的最新功能。接着,我们将深入解析MySQL的网络通信机制,包括协议、连接管理、数据传输等,让
- 497次学习
-
- JavaScript正则表达式基础与实战
- 在任何一门编程语言中,正则表达式,都是一项重要的知识,它提供了高效的字符串匹配与捕获机制,可以极大的简化程序设计。
- 487次学习
-
- 从零制作响应式网站—Grid布局
- 本系列教程将展示从零制作一个假想的网络科技公司官网,分为导航,轮播,关于我们,成功案例,服务流程,团队介绍,数据部分,公司动态,底部信息等内容区块。网站整体采用CSSGrid布局,支持响应式,有流畅过渡和展现动画。
- 484次学习
-
- 谱乐AI
- 谱乐AI是由青岛艾夫斯科技有限公司开发的AI音乐生成工具,采用Suno和Udio模型,支持多种音乐风格的创作。访问https://yourmusic.fun/,体验智能作曲与编曲,个性化定制音乐,提升创作效率。
- 2次使用
-
- Vozo AI
- 探索Vozo AI,一款功能强大的在线AI视频换脸工具,支持跨性别、年龄和肤色换脸,适用于广告本地化、电影制作和创意内容创作,提升您的视频制作效率和效果。
- 2次使用
-
- AIGAZOU-AI图像生成
- AIGAZOU是一款先进的免费AI图像生成工具,无需登录即可使用,支持中文提示词,生成高清图像。适用于设计、内容创作、商业和艺术领域,提供自动提示词、专家模式等多种功能。
- 2次使用
-
- Raphael AI
- 探索Raphael AI,一款由Flux.1 Dev支持的免费AI图像生成器,无需登录即可无限生成高质量图像。支持多种风格,快速生成,保护隐私,适用于艺术创作、商业设计等多种场景。
- 2次使用
-
- Canva可画AI生图
- Canva可画AI生图利用先进AI技术,根据用户输入的文字描述生成高质量图片和插画。适用于设计师、创业者、自由职业者和市场营销人员,提供便捷、高效、多样化的视觉素材生成服务,满足不同需求。
- 1次使用
-
- GoLand调式动态执行代码
- 2023-01-13 502浏览
-
- 用Nginx反向代理部署go写的网站。
- 2023-01-17 502浏览
-
- Golang取得代码运行时间的问题
- 2023-02-24 501浏览
-
- 请问 go 代码如何实现在代码改动后不需要Ctrl+c,然后重新 go run *.go 文件?
- 2023-01-08 501浏览
-
- 如何从同一个 io.Reader 读取多次
- 2023-04-11 501浏览